WordPress custom post types provide powerful flexibility for organizing specialized content, but their true potential is unlocked when integrated into a cohesive internal linking strategy. This guide explores how to establish effective linking rules specifically for custom post types, enhancing both user experience and SEO performance.
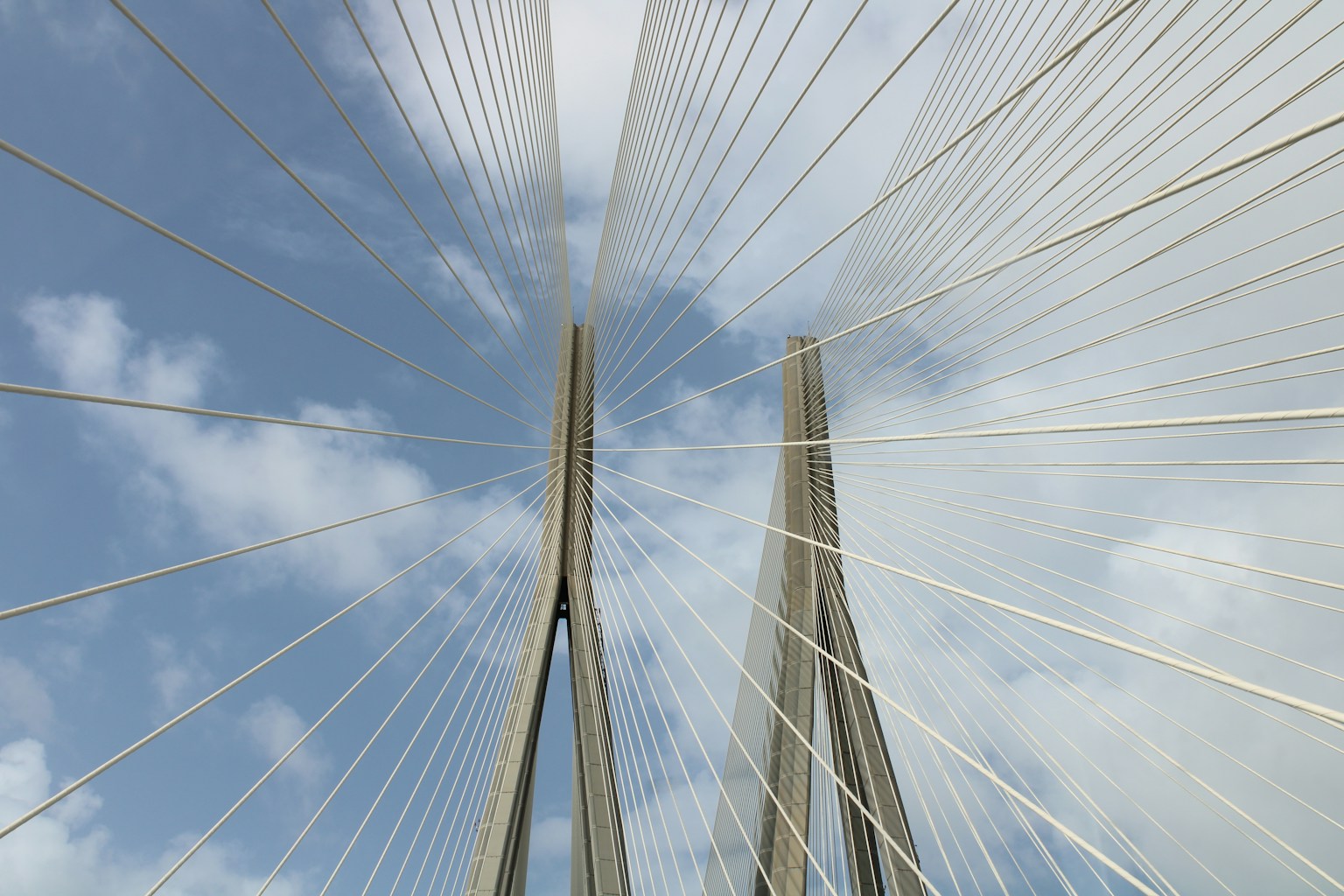
Why Custom Post Types Need Special Linking Considerations
Custom post types (CPTs) represent specialized content that often exists outside WordPress’s standard posting hierarchy. Without intentional linking strategies, these valuable content assets can become isolated from your site’s main content, reducing their visibility to both users and search engines.
According to a study by Sistrix, content that sits more than three clicks from your homepage receives significantly less search visibility. For custom post types—which frequently exist in their own silos—strategic internal linking becomes crucial for search performance.
Understanding the Technical Foundation
Custom Post Type Registration Considerations
When registering custom post types, several parameters influence how they can be linked throughout your site:
register_post_type('product',
array(
'public' => true,
'has_archive' => true,
'hierarchical' => false,
'rewrite' => array('slug' => 'products'),
'supports' => array('title', 'editor', 'thumbnail', 'excerpt', 'custom-fields')
)
);
Key parameters affecting linking include:
- has_archive: Determines whether the post type has a dedicated archive page
- hierarchical: Affects whether the post type can have parent-child relationships
- rewrite: Controls URL structure, which impacts linking patterns
- supports: Features like ‘custom-fields’ enable metadata for advanced linking rules
Implementing Custom Post Type Linking Strategies
1. Taxonomy-Based Linking Rules
One of the most effective approaches for custom post types involves utilizing custom taxonomies to establish content relationships that drive automated linking.
// Register custom taxonomy for products
register_taxonomy(
'product_category',
'product',
array(
'hierarchical' => true,
'label' => 'Product Categories',
'query_var' => true,
'rewrite' => array('slug' => 'product-category')
)
);
With taxonomies established, you can create linking rules that connect:
- Posts within the same taxonomy term
- Posts across related taxonomy terms
- Custom post types to standard posts sharing the same taxonomy
2. Implementing Automated Contextual Links
For contextual internal linking between custom post types, automated tools provide significant efficiency. The Linkify Pro plugin stands out for its ability to handle custom post types with sophisticated linking rules.
When configuring automated linking for custom post types, focus on:
Content Relevance Thresholds
Set appropriate similarity thresholds based on content specificity:
- Higher thresholds (0.3-0.4) for technical or niche custom post types
- Lower thresholds (0.2-0.3) for general informational custom post types
Cross-Type Linking Rules
Establish rules for how different post types should link to each other:
function custom_linkify_post_types($supported_types) {
$supported_types[] = 'product';
$supported_types[] = 'case_study';
return $supported_types;
}
add_filter('linkify_post_types_supported', 'custom_linkify_post_types');
Link Position Configuration
Custom post types often have unique layouts requiring specific link positioning:
function custom_link_positions($positions, $post_type) {
if ($post_type == 'product') {
return array('description', 'features', 'related');
}
return $positions;
}
add_filter('linkify_link_positions', 'custom_link_positions', 10, 2);
3. Creating Programmatic Linking Rules with WP_Query
For more complex relationships, custom queries can establish sophisticated linking connections:
function get_related_custom_posts($post_id, $post_type, $limit = 5) {
$current_post_terms = wp_get_post_terms($post_id, 'product_category', array('fields' => 'ids'));
if (empty($current_post_terms)) {
return false;
}
$args = array(
'post_type' => $post_type,
'posts_per_page' => $limit,
'post__not_in' => array($post_id),
'tax_query' => array(
array(
'taxonomy' => 'product_category',
'field' => 'id',
'terms' => $current_post_terms
)
)
);
return new WP_Query($args);
}
This function can be used to generate related content blocks that link between custom post types sharing taxonomy terms.
4. Meta Field-Based Linking
For maximum control, custom fields can establish explicit relationships between posts:
function link_posts_via_meta() {
// Get current custom post type ID
$post_id = get_the_ID();
// Get linked posts from meta field
$linked_posts = get_post_meta($post_id, 'related_resources', true);
if (!empty($linked_posts)) {
echo '<div class="related-resources">';
echo '<h3>Related Resources</h3>';
echo '<ul>';
foreach ($linked_posts as $linked_post_id) {
$linked_post = get_post($linked_post_id);
echo '<li><a href="' . get_permalink($linked_post_id) . '">' .
$linked_post->post_title . '</a></li>';
}
echo '</ul>';
echo '</div>';
}
}
This approach works particularly well with Advanced Custom Fields’ relationship field or similar tools.
Creating a Custom Post Type Link Management Interface
For content teams managing relationships between custom post types, a custom admin interface can dramatically improve workflow efficiency:
function custom_post_type_relationships_metabox() {
add_meta_box(
'cpt_relationships',
'Related Content',
'render_cpt_relationships_metabox',
'product', // your custom post type
'side',
'default'
);
}
add_action('add_meta_boxes', 'custom_post_type_relationships_metabox');
function render_cpt_relationships_metabox($post) {
wp_nonce_field('cpt_relationships_nonce', 'cpt_relationships_nonce');
$related_posts = get_post_meta($post->ID, 'related_posts', true);
$query = new WP_Query(array(
'post_type' => array('post', 'case_study'),
'posts_per_page' => -1,
'orderby' => 'title',
'order' => 'ASC'
));
if ($query->have_posts()) {
echo '<select name="related_posts[]" multiple style="width:100%; height:150px;">';
while ($query->have_posts()) {
$query->the_post();
$selected = (is_array($related_posts) && in_array(get_the_ID(), $related_posts)) ? 'selected' : '';
echo '<option value="' . get_the_ID() . '" ' . $selected . '>' . get_the_title() . '</option>';
}
echo '</select>';
echo '<p class="description">Hold Ctrl/Cmd to select multiple items</p>';
wp_reset_postdata();
}
}
function save_cpt_relationships($post_id) {
if (!isset($_POST['cpt_relationships_nonce']) ||
!wp_verify_nonce($_POST['cpt_relationships_nonce'], 'cpt_relationships_nonce')) {
return;
}
if (defined('DOING_AUTOSAVE') && DOING_AUTOSAVE) {
return;
}
if (isset($_POST['related_posts'])) {
update_post_meta($post_id, 'related_posts', $_POST['related_posts']);
} else {
delete_post_meta($post_id, 'related_posts');
}
}
add_action('save_post', 'save_cpt_relationships');
This interface allows content editors to explicitly define relationships between custom post types and other content, providing a user-friendly alternative to manual code implementations.
Advanced Implementation: Content Silos with Custom Post Types
For sites with multiple custom post types, content silos provide organizational structure while enhancing SEO. According to Search Engine Journal, topic clusters significantly outperform disconnected content.
To implement content silos with custom post types:
- Identify Main Pillar Content Typically, this would be main archive pages for each custom post type
- Create Hierarchical Link Structures Link supporting content to pillar pages while maintaining topical relevance
- Implement Cross-Linking Boundaries Limit cross-silo linking to prevent dilution of topical authority
function is_same_silo($post_id_1, $post_id_2) {
$post_1_terms = wp_get_post_terms($post_id_1, 'product_category', array('fields' => 'ids'));
$post_2_terms = wp_get_post_terms($post_id_2, 'product_category', array('fields' => 'ids'));
return array_intersect($post_1_terms, $post_2_terms);
}
function filter_contextual_links($should_link, $source_id, $target_id) {
// Allow linking if posts share a taxonomy term (same silo)
if (is_same_silo($source_id, $target_id)) {
return true;
}
// For cross-silo linking, only allow if target is a pillar page
$target_post = get_post($target_id);
if ($target_post->post_type == 'pillar_page') {
return true;
}
// Otherwise prevent cross-silo linking
return false;
}
add_filter('linkify_should_create_link', 'filter_contextual_links', 10, 3);
Measuring and Optimizing Custom Post Type Link Performance
Implementation is only the beginning—ongoing measurement and optimization are crucial for maximizing impact.
Key Metrics to Track
- Internal PageRank Distribution Tools like Screaming Frog can help visualize how link equity flows between custom post types
- User Navigation Patterns Analyze how visitors navigate between different post types using Google Analytics’ User Flow reports
- Engagement Metrics by Post Type Compare bounce rates, time on page, and conversion rates across post types to identify linking opportunities
- Crawl Depth Analysis Ensure custom post types aren’t buried too deep in your site architecture
Optimization Strategies
Based on performance data, consider these optimization approaches:
- Adjust Similarity Thresholds If links aren’t relevant enough, increase thresholds; if too few links are generated, decrease thresholds
- Create Direct Pathways for Underperforming Content Add prominent links from high-authority pages to important but underperforming custom post types
- Review and Refine Taxonomy Relationships Taxonomies drive many automated linking systems; refining your taxonomy structure often improves linking relevance
- Implement A/B Testing for Link Placement Test different link positions and formats to identify what drives the most engagement for each custom post type
Real-World Application: E-Commerce Product CPT Case Study
A specialty food e-commerce site implemented the following custom post type linking strategy for their product CPT:
- Created custom taxonomies for ingredients, dietary preferences, and meal types
- Implemented automated contextual linking between recipes (standard posts) and products (custom post type)
- Developed a custom “used in these recipes” block for product pages
- Created content silos based on dietary preference taxonomies
The results after six months included:
- 47% increase in organic traffic to product pages
- 28% reduction in bounce rate for product pages
- 23% increase in average order value when users navigated from recipes to products
- 15% improvement in average page load time due to more efficient crawling
According to site analytics, the most significant improvement came from establishing bidirectional relationships between recipe content and product pages, with visitors frequently navigating between these previously disconnected content types.
Best Practices and Common Pitfalls
Best Practices:
- Maintain Consistent URL Structures Consistent URL patterns make internal linking more predictable and maintainable
- Use descriptive anchor text Especially important for custom post types that may have less inherent context
- Balance automated and manual linking Automation provides scale while manual linking ensures strategic placement for high-priority pages
- Index custom post type taxonomies Ensuring taxonomies are indexable creates additional pathways for search engines to discover your custom post types
- Implement regular link audits As content evolves, regular audits ensure linking strategies remain effective
Common Pitfalls:
- Over-optimizing anchor text Repeating the same keywords in links can trigger spam filters; aim for natural variation
- Creating isolated content islands Some custom post types end up completely disconnected from main content; always create pathways between content types
- Ignoring mobile usability Ensure link placement works effectively on mobile devices where content displays differently
- Neglecting analytics Without measurement, you can’t determine if your linking strategy is effective
Conclusion
Custom post types provide powerful content organization capabilities, but their value is maximized only when integrated into a cohesive internal linking strategy. By implementing the technical approaches outlined in this guide—from taxonomy-based linking to custom meta field relationships—you can create a seamless content ecosystem that benefits both users and search engines.
Whether you choose automated tools like Linkify Pro or custom-coded solutions, the principles remain the same: create logical, user-focused content relationships that enhance navigation and topical authority while maintaining distinct content silos where appropriate.
The most effective custom post type linking strategies balance technical implementation with content strategy, creating an intuitive user experience while optimizing for search visibility.